SEO (Search Engine Optimization) is an essential component of digital marketing, enabling businesses to improve their online visibility and attract organic traffic. However, keeping track of SEO performance requires continuous monitoring, which can be time-consuming and prone to human error when done manually.
This is where automation comes into play. By using Python and APIs, businesses can streamline the SEO reporting process, ensuring accurate, up-to-date insights with minimal effort.
This guide will cover everything you need to know about automating SEO reporting, from setting up Python and integrating APIs to extracting valuable SEO data and automating report generation. By the end of this blog, you will be equipped with the knowledge to build your own automated SEO reporting system, saving time and improving efficiency.
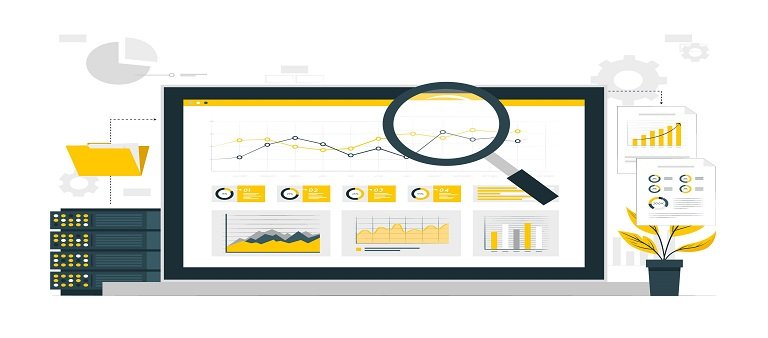
Why Automate SEO Reporting?
SEO reporting is a critical process for digital marketers, website owners, and businesses that want to improve their search engine rankings. However, manually collecting, analyzing, and presenting SEO data from multiple sources can be incredibly time-consuming and prone to errors. Automating SEO reporting helps streamline this process, ensuring efficiency, accuracy, and real-time insights.
Below are some of the key reasons why automating SEO reports is beneficial:
1. Time-Saving: Eliminate Manual Data Collection
SEO analysis requires gathering data from multiple sources such as Google Analytics, Google Search Console, Ahrefs, Moz, and SEMrush. Manually logging into each platform, exporting data, and compiling reports can take several hours or even days, especially for large-scale projects.
With automation, this entire process can be done in minutes or even seconds. Instead of spending time on repetitive tasks, you can focus on strategic decision-making, content optimization, and SEO improvements.
Example of Time Savings with Automation:
- Manual Approach: Logging into each SEO tool, downloading CSV files, merging data, and creating charts in Excel = 3-5 hours per week
- Automated Approach: Running a Python script that fetches data, processes it, and generates a report = 5-10 minutes per week
By automating SEO reporting, teams and businesses save hundreds of hours per year, allowing them to scale operations efficiently.
2. Real-Time Data Insights: Always Stay Updated
SEO is dynamic, with search engine rankings, traffic, and user behavior constantly changing. Manual reporting means delays—by the time a report is compiled, the data may already be outdated.
Automation enables real-time data retrieval and reporting, allowing businesses to:
- Monitor SEO performance continuously without waiting for monthly or quarterly reports
- Set up scheduled reports (daily, weekly, or monthly)
- Identify SEO trends faster, enabling quick adjustments to marketing strategies
- Detect technical SEO issues immediately and take corrective actions
For instance, if your website’s organic traffic suddenly drops, an automated report can notify you instantly, helping you diagnose and fix issues (such as Google algorithm updates, indexing errors, or broken links) before they impact revenue.
3. Customization & Scalability: Tailor Reports to Your Needs
Every business has different SEO goals. Some may focus on keyword rankings, while others prioritize backlink analysis, content performance, or technical SEO. Automating SEO reporting allows full customization based on business needs.
With Python, you can:
- Select specific SEO metrics you want to track (e.g., organic traffic, bounce rate, keyword rankings, backlink growth)
- Create customized client dashboard platform that present data visually in an easy-to-understand format
- Scale SEO reporting across multiple websites and clients without extra manual effort
For digital agencies managing multiple clients, automated reporting is a game-changer. Instead of manually preparing separate reports for each client, Python scripts can fetch data for dozens of clients at once, apply filters, and generate customized reports automatically.
4. Error Reduction: Ensure Accuracy & Consistency
Manual data entry is prone to errors—copy-pasting mistakes, missing data, and formatting inconsistencies can lead to inaccurate reports. Automation minimizes human intervention, ensuring reliable and consistent reporting.
Common errors in manual SEO reporting:
❌ Misaligned data when pulling metrics from multiple sources
❌ Missed updates when team members forget to refresh reports
❌ Duplicate or incorrect entries due to human error
Automation eliminates these problems by ensuring:
✅ Accurate data extraction directly from SEO tools
✅ Consistent formatting for easy comparison of metrics
✅ Error-free analysis with Python scripts validating data integrity
For businesses that rely on SEO for revenue, precise reporting is critical. Even a small error in ranking data or traffic numbers can mislead decision-making and impact marketing strategies.
Essential Tools & Libraries for SEO Report Automation
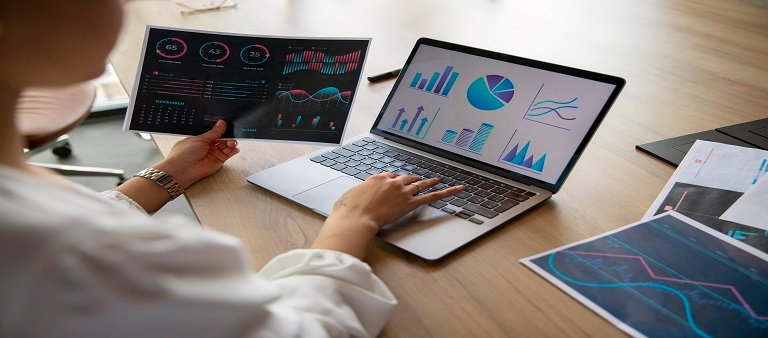
To successfully automate SEO reporting, you need the right set of tools and technologies. The two key components of automation are:
- Python Libraries – for data extraction, processing, and visualization
- SEO APIs – for retrieving data from SEO platforms like Google Analytics and Ahrefs
1. Python Libraries for SEO Automation
Python is widely used for data analysis and automation due to its ease of use and extensive library support. The following Python libraries are essential for automating SEO reports:
1.1. pandas – Data Processing & Analysis
- Used to clean, structure, and manipulate large SEO datasets
- Helps organize keyword rankings, traffic metrics, and backlink data in structured formats
- Example usage:
import pandas as pd
data = {“Keyword”: [“SEO automation”, “Python SEO”], “Clicks”: [200, 150]}
df = pd.DataFrame(data)
print(df)
1.2. requests – API Data Retrieval
- Allows Python to fetch SEO data from APIs (Google Analytics, Search Console, Ahrefs, etc.)
- Example usage to get data from an API:
import requests
url = “https://api.example.com/seo_data”
response = requests.get(url)
data = response.json()
print(data)
1.3. google-auth – Authentication for Google APIs
- Used to authenticate and connect with Google services (Google Analytics, Search Console)
- Essential for retrieving organic traffic, keyword rankings, and user behavior data
1.4. matplotlib & seaborn – Data Visualization
- Used to visualize SEO performance with charts and graphs
- Helps create trend analysis charts for keyword growth, traffic changes, and backlink growth
import matplotlib.pyplot as plt
keywords = [“SEO automation”, “Python SEO”]
clicks = [200, 150]
plt.bar(keywords, clicks, color=”blue”)
plt.xlabel(“Keywords”)
plt.ylabel(“Clicks”)
plt.title(“Keyword Performance”)
plt.show()
2. APIs for Data Extraction
To automate SEO reporting, you need APIs (Application Programming Interfaces) that provide real-time SEO data. Below are the most commonly used SEO APIs:
2.1. Google Search Console API
- Provides keyword ranking data, search queries, click-through rates (CTR), and indexed pages
- Helps in monitoring SEO performance and indexing issues
- Ideal for tracking keyword rankings & impressions over time
2.2. Google Analytics API
- Fetches website traffic, user behavior, and conversion data
- Helps understand organic vs. paid traffic trends
- Useful for tracking bounce rate, average session duration, and user engagement
2.3. Ahrefs API / SEMrush API
- Provides backlink analysis, domain authority, and competitor keyword insights
- Helps in monitoring backlink profile growth
- Useful for detecting lost backlinks and link-building opportunities
2.4. Moz API
- Offers insights into Domain Authority (DA) and Page Authority (PA)
- Helps measure website strength compared to competitors
By integrating these APIs with Python scripts, you can automate SEO data retrieval, processing, and reporting, making SEO management more efficient and data-driven.
Step-by-Step Guide to Automating SEO Reporting
Step 1: Setting Up API Access for SEO Tools
To access data from SEO tools, you first need to authenticate and connect to their APIs.
1.1 Enabling Google Search Console API
- Go to Google Cloud Console.
- Create a new project and enable Google Search Console API.
- Navigate to API & Services > Credentials and create an OAuth 2.0 Client ID.
- Download the JSON key file, which will be used to authenticate API requests.
1.2 Installing Python Libraries
Before proceeding, install the required Python libraries using pip:
pip install pandas requests google-auth google-auth-oauthlib google-auth-httplib2 matplotlib seaborn
Once installed, you’re ready to start fetching SEO data.
Step 2: Extracting Data from Google Search Console
Google Search Console (GSC) provides valuable insights into how your website appears in search results. The following script will extract top search queries from GSC.
import requests
import json
import pandas as pd
from google.oauth2 import service_account
# Load API credentials
SERVICE_ACCOUNT_FILE = “path/to/json-key-file.json”
SCOPES = [“https://www.googleapis.com/auth/webmasters.readonly”]
credentials = service_account.Credentials.from_service_account_file(
SERVICE_ACCOUNT_FILE, scopes=SCOPES
)
# Define API request parameters
site_url = “https://yourwebsite.com”
api_url = f”https://www.googleapis.com/webmasters/v3/sites/{site_url}/searchAnalytics/query”
# Define the request body
request_body = {
“startDate”: “2024-01-01”,
“endDate”: “2024-03-01”,
“dimensions”: [“query”],
“rowLimit”: 10
}
# Make API call
response = requests.post(api_url, json=request_body, headers={“Authorization”: f”Bearer {credentials.token}”})
data = response.json()
# Convert API response to DataFrame
df = pd.DataFrame(data[‘rows’])
print(df)
This script retrieves the top-performing search queries from Google Search Console.
Step 3: Fetching Traffic Data from Google Analytics
Google Analytics provides insights into website traffic, user engagement, and conversion rates. The following script extracts user sessions and traffic sources:
from googleapiclient.discovery import build
# Authenticate
credentials = service_account.Credentials.from_service_account_file(
SERVICE_ACCOUNT_FILE, scopes=[“https://www.googleapis.com/auth/analytics.readonly”]
)
analytics = build(“analyticsreporting”, “v4”, credentials=credentials)
# Fetch data
response = analytics.reports().batchGet(
body={
“reportRequests”: [{
“viewId”: “YOUR_VIEW_ID”,
“dateRanges”: [{“startDate”: “30daysAgo”, “endDate”: “today”}],
“metrics”: [{“expression”: “ga:sessions”}, {“expression”: “ga:users”}],
“dimensions”: [{“name”: “ga:sourceMedium”}]
}]
}
).execute()
print(json.dumps(response, indent=2))
This script provides traffic source insights, which are essential for evaluating marketing performance.
Step 4: Extracting Backlink Data Using Ahrefs API
Backlink monitoring is a key aspect of SEO. Ahrefs API helps in tracking link-building efforts:
AHREFS_API_KEY = “your_ahrefs_api_key”
target_url = “https://yourwebsite.com”
api_url = f”https://apiv2.ahrefs.com?token={AHREFS_API_KEY}&target={target_url}&mode=exact&output=json&from=backlinks”
response = requests.get(api_url)
backlink_data = response.json()
print(backlink_data)
This script retrieves backlinks pointing to your website.
Step 5: Automating Data Visualization
Once the data is extracted, it can be visualized for better interpretation.
Visualizing Keyword Performance
import matplotlib.pyplot as plt
import seaborn as sns
# Sample keyword data
df = pd.DataFrame({
“query”: [“seo automation”, “python for seo”, “automate seo reporting”],
“clicks”: [250, 180, 300],
“impressions”: [5000, 4000, 6000]
})
# Plot impressions vs. clicks
plt.figure(figsize=(10,5))
sns.barplot(x=”query”, y=”clicks”, data=df, color=”blue”, label=”Clicks”)
sns.barplot(x=”query”, y=”impressions”, data=df, color=”lightblue”, label=”Impressions”)
plt.xlabel(“Search Queries”)
plt.ylabel(“Performance Metrics”)
plt.title(“Keyword Performance”)
plt.legend()
plt.show()
Step 6: Automating Email Report Delivery
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
message = MIMEMultipart()
message[“From”] = “your_email@gmail.com”
message[“To”] = “recipient@example.com”
message[“Subject”] = “Automated SEO Report”
message.attach(MIMEText(“Attached is your latest SEO report.”, “plain”))
server = smtplib.SMTP(“smtp.gmail.com”, 587)
server.starttls()
server.login(“your_email@gmail.com”, “your_password”)
server.sendmail(“your_email@gmail.com”, “recipient@example.com”, message.as_string())
server.quit()
Conclusion
By automating SEO reporting, businesses can enhance efficiency, improve decision-making, and gain deeper insights into website performance. Using Python and APIs, you can create a fully automated system for tracking SEO metrics.
Start automating today and stay ahead in SEO!